XML Conversion Using Python in 2024
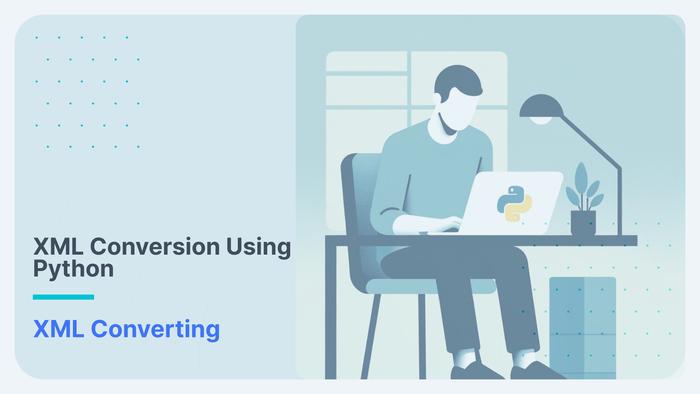
XML (eXtensible Markup Language) is a common data format for data exchange and used in various industry data standards such as ISO 20022, HL7, ACORD just to name a few. However, for data analysis purposes the data locked away in XML needs to be converted to a more suitable format, typically a relational database or CSV.
One option to convert XML is Python. Python offers several practical methods and tools for handling XML. Among these, ElementTree stands out for its simplicity, being part of the standard library and offering an easy-to-use API for XML operations. lxml is favored for performance-sensitive tasks, leveraging the libxml2 and libxslt libraries for fast processing.
xmltodict simplifies XML interaction by converting documents into Python dictionaries, making data manipulation more intuitive. Choosing between these libraries depends on the project’s specific needs, such as performance, ease of use, or particular XML handling requirements.
In this document, we’ll explore the various tools and techniques for converting XML to a tabular or relational format in Python. We will also discuss the scenarios when a fully automated approach using an XML conversion tool makes more sense than hand coding a custom solution.
Use Flexter to turn XML and JSON into Valuable Insights
- 100% Automation
- 0% Coding
Popular Libraries and Methods for XML Conversion in Python
Python provides several libraries and methods suitable for XML conversion, catering to various data handling requirements. While XML is one of many formats used for data exchange and storage, its conversion into more commonly used formats like CSV can enhance data interoperability and integration.
This overview introduces key Python libraries, including lxml, ElementTree, xmltodict, BeautifulSoup, and pandas, that facilitate the conversion process. These libraries offer diverse approaches for parsing, modifying, and converting XML.
1. ElementTree API
Introduction and Basic Usage
The ElementTree API is a built-in Python library for parsing and creating XML data.
Basic usage involves importing the library, parsing an XML file, and then navigating or manipulating the tree structure.
Pros and Cons
Pros: Integrated into Python’s standard library, user-friendly, sufficient for most basic XML tasks.
Cons: Not as fast as some external libraries, lacks some advanced features like XPath support.
Input XML
1 2 3 4 5 6 7 8 9 10 |
<root> <record> <book>Da Vinci Code</book> <category>Fiction</category> </record> <record> <book>C++</book> <category>Education</category> </record> </root> |
Code Snippet
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
import xml.etree.ElementTree as ET import csv # Load and parse the XML file tree = ET.parse('/home/sonra/Desktop/book.xml') root = tree.getroot() with open('/home/sonra/Desktop/output.csv', 'w', newline='') as csvfile: csvwriter = csv.writer(csvfile) headers = ['BOOK', 'CATEGORY'] csvwriter.writerow(headers) # Iterate through each record in the XML and write to the CSV file for record in root.findall('record'): field1 = record.find('book').text field2 = record.find('category').text csvwriter.writerow([field1, field2]) |
Output
Output is a csv file containing the following;
BOOK | CATEGORY |
---|---|
Da Vinci Code | Fiction |
C++ | Education |
2. Lxml
Introduction and Basic Usage
lxml is a third-party Python library that extends the capabilities of ElementTree with additional features and improved performance. It is often used as a drop-in replacement for ElementTree due to its similar API.
Basic usage typically involves importing the library, parsing XML, and using XPath for advanced querying.
Pros and Cons
Pros: Faster parsing and better memory management, especially for large XML files. Comprehensive support for XPath, XSLT, and schema validation.
Cons: External dependency, slightly more complex than ElementTree.
Input XML
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
<University id="TUD"> <Faculty id="Engineering"> <Department id="ComputerScience"> <Course>Introduction to Programming</Course> <Course>Algorithms and Data Structures</Course> </Department> <Department id="ElectricalEngineering"> <Course>Circuit Analysis</Course> <Course>Electromagnetics</Course> <Course>Control Systems</Course> </Department> <ResearchGroup id="ArtificialIntelligence"> <Project>Machine Learning Advances</Project> <Project>Neural Network Optimization</Project> <Project>AI Ethics and Society</Project> </ResearchGroup> <ResearchGroup id="Nanotechnology"> <Project>Nano-materials Engineering</Project> <Project>Quantum Computing</Project> </ResearchGroup> </Faculty> <AdministrativeUnit id="Library"> <Service>Book Lending</Service> <Service>Research Assistance</Service> </AdministrativeUnit> <AdministrativeUnit id="IT"> <Service>Campus Network</Service> <Service>Helpdesk</Service> </AdministrativeUnit> </University> |
Code Snippet
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 |
from lxml import etree import csv data_containers = { "Faculty": [], "Department": [], "ResearchGroup": [], "AdministrativeUnit": [], "Course": [], "Project": [], "Service": [], "University": [] } def categorize_data(element, university_id=None, faculty_id=None, department_id=None, research_group_id=None, administrative_unit_id=None): element_id = element.attrib.get('id', None) # Handling for 'University' if element.tag == "University" and element_id: university_id = element_id data_containers["University"].append({ "University ID": university_id, }) # Handling for 'Faculty' elif element.tag == "Faculty" and element_id: faculty_id = element_id data_containers["Faculty"].append({ "University ID": university_id, "Faculty ID": faculty_id, }) # Handling for 'Department' elif element.tag == "Department" and element_id: department_id = element_id data_containers["Department"].append({ "University ID": university_id, "Faculty ID": faculty_id, "Department ID": department_id, }) # Handling for 'ResearchGroup' elif element.tag == "ResearchGroup" and element_id: research_group_id = element_id data_containers["ResearchGroup"].append({ "University ID": university_id, "Faculty ID": faculty_id, "Research Group ID": research_group_id, }) # Handling for 'AdministrativeUnit' elif element.tag == "AdministrativeUnit" and element_id: administrative_unit_id = element_id data_containers["AdministrativeUnit"].append({ "University ID": university_id, "Administrative Unit ID": administrative_unit_id, }) # Handling for 'Course' elif element.tag == "Course": course_name = element.text.strip() data_containers["Course"].append({ "Department ID": department_id, "Course Name": course_name, }) # Handling for 'Project' elif element.tag == "Project": project_name = element.text.strip() data_containers["Project"].append({ "Research Group ID": research_group_id, "Project Name": project_name, }) # Handling for 'Service' elif element.tag == "Service": service_name = element.text.strip() data_containers["Service"].append({ "Administrative Unit ID": administrative_unit_id, "Service Name": service_name, }) for child in element: categorize_data(child, university_id, faculty_id, department_id, research_group_id, administrative_unit_id) def write_data_to_csv(): for tag, data in data_containers.items(): if data: with open(f'{tag}.csv', 'w', newline='') as file: writer = csv.DictWriter(file, fieldnames=data[0].keys()) writer.writeheader() writer.writerows(data) print(f"Data written to {tag}.csv") def parse_and_write(xml_file): tree = etree.parse(xml_file) root = tree.getroot() categorize_data(root, root.attrib['id']) write_data_to_csv() xml_file_path = '/home/sonra/Desktop/book.xml' parse_and_write(xml_file_path) |
Output
Output consists of multiple csv files where each csv file contains each tag and its corresponding details.
AdministrativeUnit.csv
University ID | Administrative Unit ID |
---|---|
TUD | Library |
TUD | IT |
Course.csv
Department ID | Course Name |
---|---|
ComputerScience | Introduction to Programming |
ComputerScience | Algorithms and Data Structures |
ElectricalEngineering | Circuit Analysis |
ElectricalEngineering | Electromagnetics |
ElectricalEngineering | Control Systems |
Department.csv
University ID | Faculty ID | Department ID |
---|---|---|
TUD | Engineering | ComputerScience |
TUD | Engineering | ElectricalEngineering |
Faculty.csv
University ID | Faculty ID |
---|---|
TUD | Engineering |
Project.csv
Research Group ID | Project Name |
---|---|
ArtificialIntelligence | Machine Learning Advances |
ArtificialIntelligence | Neural Network Optimization |
ArtificialIntelligence | AI Ethics and Society |
Nanotechnology | Nano-materials Engineering |
Nanotechnology | Quantum Computing |
ResearchGroup.csv
University ID | Faculty ID | Research Group ID |
---|---|---|
TUD | Engineering | ArtificialIntelligence |
TUD | Engineering | Nanotechnology |
Service.csv
Administrative Unit ID | Service Name |
---|---|
Library | Book Lending |
Library | Research Assistance |
IT | Campus Network |
IT | Helpdesk |
University.csv
University ID |
---|
TUD |
3. Xmltodict
Introduction and Basic Usage
xmltodict is a Python library that makes working with XML feel like working with JSON by converting XML into Python dictionaries.
Basic usage includes importing the library and using it to parse XML files, allowing for easy access to elements as dictionary keys.
Pros and Cons
Pros: Simplifies XML data structure, making it more Pythonic and easier to understand and manipulate.
Cons: Might not be suitable for highly complex XML structures or when preserving the order of elements is critical.
Input XML
1 2 3 4 5 6 7 8 9 10 |
<root> <record> <book>Da Vinci Code</book> <category>Fiction</category> </record> <record> <book>C++</book> <category>Education</category> </record> </root> |
Code Snippet
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
import xmltodict import csv # Read and parse the XML file with open('/home/sonra/Desktop/book.xml', 'r') as file: xml_data = file.read() data_dict = xmltodict.parse(xml_data) # Open a CSV file for writing with open('/home/sonra/Desktop/output2.csv', 'w', newline='') as csvfile: csvwriter = csv.writer(csvfile) # Define the headers headers = ['BOOK', 'CATEGORY'] csvwriter.writerow(headers) # Extract and write data to CSV for record in data_dict['root']['record']: book = record['book'] category = record['category'] csvwriter.writerow([book, category]) |
Output
Output is a csv file containing the following;
BOOK | CATEGORY |
---|---|
Da Vinci Code | Fiction |
C++ | Education |
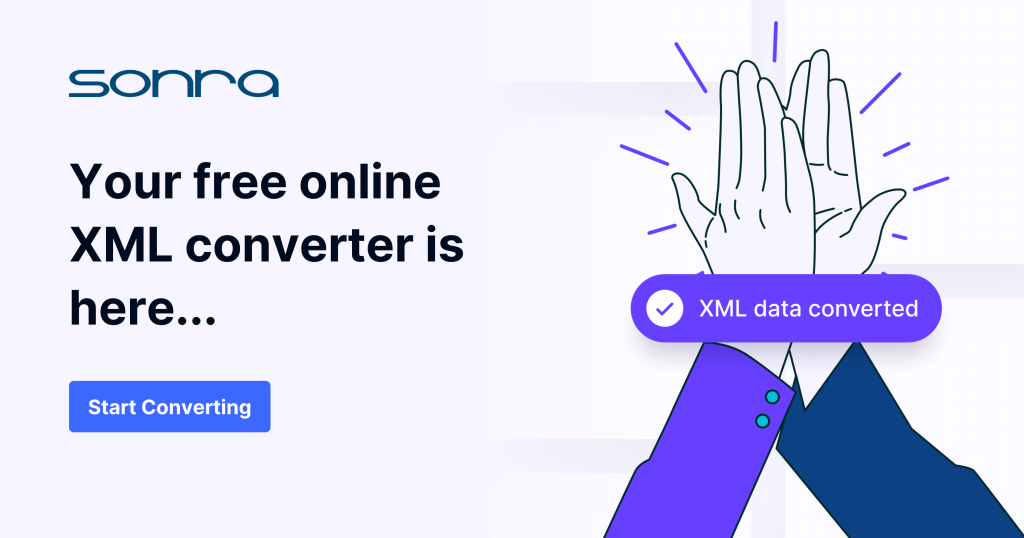
4. Beautiful Soup
Introduction and Basic Usage
Beautiful Soup is a Python library for parsing HTML and XML documents, commonly used for web scraping.
Basic usage involves importing the library, parsing XML data, and then using its intuitive API to navigate and search the document.
Provides a more intuitive interface for navigating and searching the document tree.
Not as fast as lxml, but easier for extracting data from irregular XML structures.
Pros and Cons
Pros: User-friendly and ideal for parsing and extracting data from irregularly structured XML, like HTML.
Cons: Slower compared to lxml, more suitable for web data scraping than large-scale XML data processing.
Input XML
1 2 3 4 5 6 7 8 9 10 |
<root> <record> <book>Da Vinci Code</book> <category>Fiction</category> </record> <record> <book>C++</book> <category>Education</category> </record> </root> |
Code Snippet
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
from bs4 import BeautifulSoup import csv # Read and parse the XML file with open('/home/sonra/Desktop/book.xml', 'r') as file: xml_data = file.read() soup = BeautifulSoup(xml_data, 'lxml') # Open a CSV file for writing with open('/home/sonra/Desktop/output3.csv', 'w', newline='') as csvfile: csvwriter = csv.writer(csvfile) # Define the headers headers = ['BOOK', 'CATEGORY'] csvwriter.writerow(headers) # Extract and write data to CSV for record in soup.find_all('record'): book = record.find('book').text category = record.find('category').text csvwriter.writerow([book, category]) |
Output
Output is a csv file containing the following;
BOOK | CATEGORY |
---|---|
Da Vinci Code | Fiction |
C++ | Education |
5. Pandas
Introduction and Basic Usage
Pandas is a data manipulation and analysis library that can also read XML data directly into DataFrames.
Basic usage includes importing Pandas and using the read_xml function to convert XML into a structured DataFrame.
Pros and Cons
Pros: Integrates XML data seamlessly into data analysis workflows, easy conversion to tabular format.
Cons: Not as versatile for XML manipulation as other libraries, more suitable for data analysis than general XML processing.
Sample Code Snippet
1 2 3 4 |
import pandas as pd df = pd.read_xml('example.xml') print(df) |
Each library offers unique features and caters to different needs in XML processing, making them valuable tools in a Python developer’s toolkit for handling XML data.
Tools for automatically converting XML
Advantages of an automated approach
While Python libraries offer flexibility when it comes to XML conversion projects, XML converters fully automate the whole conversion process. These tools offer the following advantages:
- Increased Efficiency: These systems significantly shorten the time required to complete projects, enabling quicker transition from development to deployment. The automation speeds up the processing, making it possible to get projects up and running in less time.
- Adaptability to Data Volume: Whether dealing with large datasets or smaller volumes, these tools can adjust their operations to handle any size efficiently. This flexibility ensures that both expansive and modest projects can be managed effectively.
- Simplified Complexity Management: Complex data formats, including various types of XML and XSD, can be easily managed. This capability makes navigating through intricate data structures less daunting and more straightforward.
- Reduced Dependency on Expertise: The intuitive design of these tools lessens the need for specialized knowledge in XML, opening up the process to a broader audience. This aspect makes it more user-friendly and less reliant on extensive training.
- Minimal Code Adjustments: The need for frequent code modifications in response to project changes is drastically reduced. This stability not only simplifies maintenance but also enhances the overall manageability of the codebase.
- Decreased Risk of Setbacks: By minimizing the likelihood of encountering delays and overspending, these tools help ensure that projects progress smoothly and stay within budget, leading to more predictable outcomes.
- Quicker Data Preparation: The automation of the conversion process, from analyzing data to creating schemas and mapping, means data is prepared for use more rapidly. This efficiency aids in making timely decisions based on the processed data.
- Consistent and Reliable Data Handling: The automated approach ensures a lower risk of human error, maintaining a consistent quality of data processing. This reliability is crucial for upholding data integrity and accuracy.
- Ease of Handling Complex Data: With user-friendly interfaces, these tools make processing complex data formats more accessible, reducing the need for rare XML expertise. This ease of use helps to simplify tasks that were previously considered challenging.
Choosing between Python coding and automated XML conversion
Choosing between Python coding and using an automated XML conversion tool for dealing with XML data really comes down to what your project’s specific needs are, especially considering the complexity of your XML files and how much customization the conversion process requires.
Although Python has a large library ecosystem and strong community support for converting XML files, facilitating custom solutions and straightforward integration across diverse data processing frameworks, it is not without its drawbacks. The challenges include the possibility of performance problems while processing huge files, a long development time, and a steep learning curve.
The potential of automated XML conversion solutions to simplify the conversion process is one of its many benefits. These tools perform exceptionally well in situations requiring quick XML conversion allowing organisations to transform massive amounts of XML files with minimal manual labour and coding.
Because of the user-friendly design of these tools, even those with little to no programming knowledge can convert XML files. Automating repeated processes not only improves the accuracy of the data produced by lowering the possibility of human error but also speeds up the conversion process.
Additionally, the characteristics of automated tools make scalability easy, enabling them to handle projects of different sizes without requiring extra programming. This makes them a good resource for businesses trying to effectively and precisely handle their XML data conversion requirements. That is where Flexter comes in, embodying these advantages and offering a specialised solution for streamlined XML data management.
In summary, an automated XML conversion solution is a good fit if you say yes to one or more of these questions.
- Are your XML documents complex, e.g. are they based on an industry data standard, come with one or more XSDs, or have more than 50 XPaths (XML elements)?
- Do you have large volumes of XML data?
- Do you have many different types of XML documents and XML schemas?
- Do you have very large XML files?
- Do you lack XML skills such as XQuery, XPath, XSLT on your engineering team?
For simple and ad hoc conversion requirements a manual coding approach is more suitable.
Flexter – Your Solution for Complex XML Conversion:
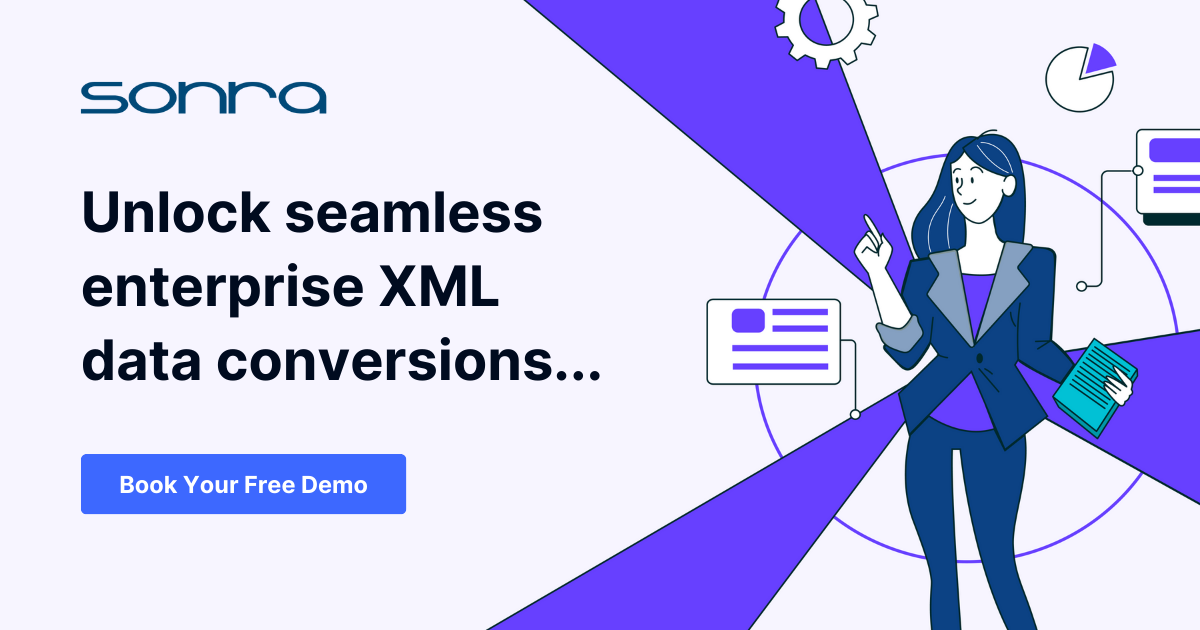
For projects facing challenges with complex XML structures, substantial file sizes, or tight deadlines, Flexter is a great XML converter. Its free online version allows for an initial evaluation of the tool’s capabilities in simplifying XML conversion.
Flexter’s team is available to discuss specific requirements, ensuring a streamlined and effective conversion process, contrasting with the labour intensive approach of Python scripting.